First feeling
Learning a new langage feels ecxiting and scary at the same time. You are excited because you are going to learn something new, that’s by it self is very ecxiting regardless of what you are going to learn. And at the same time, it is scary because you have to get out of your confort zone. However, this time, i felt more excited than when i initially decided to learn javascript. I guess my level of confidence went up since the beggining of my coder journey.
Following are my intial notes after a week of diving into Python @LambdaSchool. I will be using Javascript as point of comparaison where relevant.
Structuring the code
Variables
The first thing i have noticed, is the absence of varariables label in Python. There is no need to label your variables
with const
, var
or let
. You just give a variable name, and its initial value.
The type of the intial value assigned will define how the variable can be used later, mostly if it is mutable or not.
1# Mutable varialble (string)2my_variable = "initial value" # let my_variable = "initial value" in js34# Non Mytable variable (tuple)5tuple_variable = ("some data") # const tuple_variable = ["some data"]
Comments
Single line comment in Python start with the character #
.
1# this is a single line comment2# Equivalent to `//` in js
Console.log
This is straight forward
1print("something") # console.log("something")
Print()
can also take several formating parameters such as the one below
1print(f"Received variable {variable}") # console.log(`Received variable ${variable}`)
Operators
As an overall appraoch, Python seems to be designed to be as close as possible to english words
.
Therefore in many cases, symbols will be replaced by plain english words.
Also worth noting that conditional test else if
is shortened to elif
1# Logical operators in python2if A and B: # if (A && B) {}3elif A or B: # else if ( A || B) {}4elif A not B: # else if ( A != B) {}5else: # else {}
Python’s membership operators test the presence of a given data inside a sequence (strings, lists, …) Following are the two main onces
1a = 102b = 203list = [1, 4, 5, 10 ]45if a in list: # so easy to read and remember :)6 print("A is present in the list") # Console.log("A is present in the list")7if b not in list:8 print("B is not present in the list") # Console.log("B is not present in the list")
Stronger types (int, string, float, )
Python is a typed langage, it requires that your data types are in synch before executing it.
For example, a string
and an integer
cannot be concanated unless the integer is explicitly converted into a string.
Which is not required in Javascript.
1integer = 4 # const loop = 42string = "Number of wheels" # const string = "Number of wheels"34# the integer is converted first with str(). in JS const phrase = string + integer;5phrase = string + str(integer)
List (array?)
List
in Python are similar to array
in JavaScript.
1# Python list2my_fruits = ["orange", "apple", "pear"] # const my_fruits = ["orange", "apple", "pear"]
Dictionnary (Object?)
As for Dictionary
, the closest thing in JavaScript are objects
1# Python dictionary2my_fruits = {3 "name": "apple",4 "color" "red",5 "prce": "106 } # same in js but with a lable (const, var, let) before the variable name
Tuple (Imutable list)
Tuple
are like List
, but with the following specificities.
- The data intialisation is wrapped between two parentheses
()
, not square brackets[]
Tuples
are not mutable. It can not be changed once initialised
1# Python typle2my_fruits = ("orange", "apple", "pear")
Functions
Column, Semi-column, Indentation
Functions bloc in Python, are NOT wrapped inside curly brackets {}
. It uses a combinaison of column :
and indentation after the column, to define the start/end of a bloc of code. Its advised to use 4 spaces.
Tabs can be used as well but the entire script must be consistent (not mixin spaces and Tabs).
The key word function
in js is also replace by key word def
in Python.
Finally, there is no need to finish each line of code with a semi-colun ;
as we would do in Javascript
1# Python function2def my_function (a, b): # the column define the beggining of the bloc3 return a + b # each line of code inside the function must have the same indentation4# }
Passing as reference vs Value
Argument in Python can be passed as reference, or as value. Passing a value will make a copy of the original data, and that copy will eventually be mutated by the function. On the other hand, passing as reference send a reference of the original data (memory location) to the function. Therefore, if you try to mutate the received variable, the original data that will mutate (change).
If you are like me, being a visual
person, this illustration is quite helpfull to understand the difference.
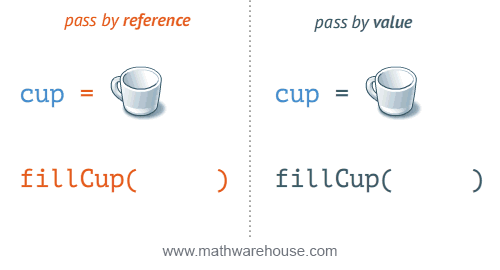
Having said that, from a pure syntaxe point of view, I could not find a way to differentiate the two approaches. My understanding is that the type of argument (mutable or not) the function receives, will define the behaviour (by reference or value). Probably more on that further down the line.
Undefined number of arguments
A cool feature in Python is to be able to write a function that accepts an arbitrary number of keyword arguments. I am not sure yet practically how it can be used/usefull, but its good to keep in mind.
1def with_arbitrary_num_of_arg(**keywords):2 for key in keywords:3 print(f"key: {key}, value: {keywords[key]}")45# Should print6# key: a, value: 127# key: b, value: 308with_arbitrary_num_of_arg(a=12, b=30)
Class
Self
The self
keyword in python class is the equivalent of this
in JavaScript class.
Inheritence (is a)
A class can inherit from a parent
classe properties and methodes by :
1. Passing the parent as argument of the child class
2. Refering to the parent in the child class constructor with the keyword super
1# Parent class2class LatLon:3 def __init__(self, lat, lon):4 self.lat = lat5 self.lon = lon67# Child class --> Waupoint (is a) LatLon8class Waypoint(LatLon): # Receiving parent class as argument9 def __init__(lat, lon, name):10 # Child class constructor with reference to its parent using `super` keyword11 super().__init__(self, lat, lon)12 self.name = name # Child class specific property
Composition (has a)
Composition is a technique to define a class that can be composed with other classes properties and methodes.
A way to identify this type of relationship to use has a
between them. For example, in below code, a Car
has a Motor
.
1# Class Motor2class Motor:3 def __init__(self, make, cc):4 self.make = make5 self.cc = cc67# Class Car8class Car:9 def __init__(self, make, model, engine):10 self.make = make11 self.model = model12 self.engine = engine1314# Instance of a Motor15honda_petrol_motor = Motor("Honda", 1799)16# Instance of Car that has a Honda motor17car = Car("Honda", "Civic", honda_petrol_motor)
Conclusion
Python feels fairly accessible when you already have some notions of coding from another langage.
I like its closeness to english word
compared to JavaScript. It makes the code more readable,
and building memory muscle faster. Additionaly, its seems to be a good choice for lower level process, such
as reading and manipulating files from the system, or accessing operating system info.
At the time of writing this article, I mostly used Python to practice writing algorithms.
That will be the subject of my next article.